Chain shape configuration. More...
#include <ChainShapeConf.hpp>
Public Member Functions | |
ChainShapeConf () | |
Default constructor. More... | |
ChainShapeConf & | Set (std::vector< Length2 > arg) |
Sets the configuration up for representing a chain of vertices as given. More... | |
ChainShapeConf & | Add (Length2 vertex) |
Adds the given vertex. More... | |
ChainShapeConf & | Transform (const Mat22 &m) noexcept |
Transforms all the vertices by the given transformation matrix. More... | |
ChildCounter | GetChildCount () const noexcept |
Gets the "child" shape count. More... | |
DistanceProxy | GetChild (ChildCounter index) const |
Gets the "child" shape at the given index. More... | |
MassData | GetMassData () const noexcept |
Gets the mass data. More... | |
ChainShapeConf & | UseVertexRadius (NonNegative< Length > value) noexcept |
Uses the given vertex radius. More... | |
ChildCounter | GetVertexCount () const noexcept |
Gets the vertex count. More... | |
Length2 | GetVertex (ChildCounter index) const |
Gets a vertex by index. More... | |
UnitVec | GetNormal (ChildCounter index) const |
Gets the normal at the given index. More... | |
![]() | |
PLAYRHO_CONSTEXPR | ShapeBuilder ()=default |
Default constructor. More... | |
PLAYRHO_CONSTEXPR | ShapeBuilder (const BaseShapeConf &value) noexcept |
Initializing constructor. More... | |
PLAYRHO_CONSTEXPR ChainShapeConf & | UseFriction (NonNegative< Real > value) noexcept |
Uses the given friction. More... | |
PLAYRHO_CONSTEXPR ChainShapeConf & | UseRestitution (Finite< Real > value) noexcept |
Uses the given restitution. More... | |
PLAYRHO_CONSTEXPR ChainShapeConf & | UseDensity (NonNegative< AreaDensity > value) noexcept |
Uses the given density. More... | |
Static Public Member Functions | |
static PLAYRHO_CONSTEXPR NonNegative< Length > | GetDefaultVertexRadius () noexcept |
Gets the default vertex radius. More... | |
Public Attributes | |
NonNegative< Length > | vertexRadius = GetDefaultVertexRadius() |
Vertex radius. More... | |
![]() | |
NonNegative< Real > | friction = NonNegative<Real>{Real{2} / Real{10}} |
Friction coefficient. More... | |
Finite< Real > | restitution = Finite<Real>{0} |
Restitution (elasticity) of the associated shape. More... | |
NonNegative< AreaDensity > | density = NonNegative<AreaDensity>{0_kgpm2} |
Area density of the associated shape. More... | |
Friends | |
bool | operator== (const ChainShapeConf &lhs, const ChainShapeConf &rhs) noexcept |
Equality operator. More... | |
bool | operator!= (const ChainShapeConf &lhs, const ChainShapeConf &rhs) noexcept |
Inequality operator. More... | |
Detailed Description
Chain shape configuration.
A chain shape is a free form sequence of line segments. The chain has two-sided collision, so you can use inside and outside collision. Therefore, you may use any winding order. Since there may be many vertices, they are allocated on the memory heap.
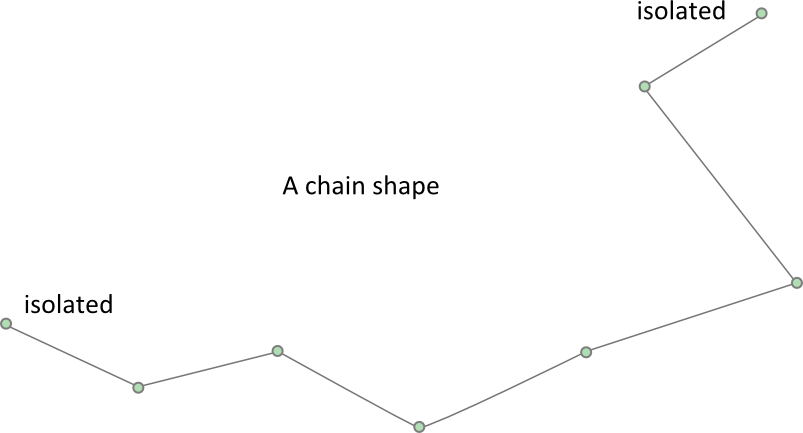
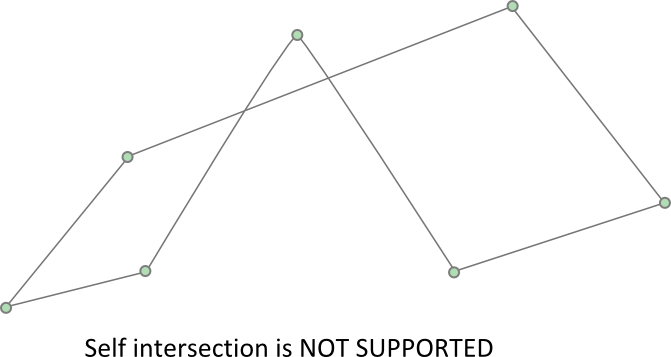
- Warning
- The chain will not collide properly if there are self-intersections.
Definition at line 49 of file ChainShapeConf.hpp.
Constructor & Destructor Documentation
◆ ChainShapeConf()
|
default |
Default constructor.
Member Function Documentation
◆ GetDefaultVertexRadius()
|
inlinestaticnoexcept |
Gets the default vertex radius.
Definition at line 53 of file ChainShapeConf.hpp.
◆ Set()
ChainShapeConf & playrho::d2::ChainShapeConf::Set | ( | std::vector< Length2 > | arg | ) |
Sets the configuration up for representing a chain of vertices as given.
Definition at line 51 of file ChainShapeConf.cpp.
◆ Add()
ChainShapeConf & playrho::d2::ChainShapeConf::Add | ( | Length2 | vertex | ) |
Adds the given vertex.
Definition at line 99 of file ChainShapeConf.cpp.
◆ Transform()
|
noexcept |
Transforms all the vertices by the given transformation matrix.
- Note
- This updates the normals too.
Definition at line 90 of file ChainShapeConf.cpp.
◆ GetChildCount()
|
inlinenoexcept |
Gets the "child" shape count.
Definition at line 73 of file ChainShapeConf.hpp.
◆ GetChild()
DistanceProxy playrho::d2::ChainShapeConf::GetChild | ( | ChildCounter | index | ) | const |
Gets the "child" shape at the given index.
Definition at line 157 of file ChainShapeConf.cpp.
◆ GetMassData()
|
noexcept |
Gets the mass data.
Definition at line 116 of file ChainShapeConf.cpp.
◆ UseVertexRadius()
|
inlinenoexcept |
Uses the given vertex radius.
Definition at line 145 of file ChainShapeConf.hpp.
◆ GetVertexCount()
|
inlinenoexcept |
Gets the vertex count.
Definition at line 90 of file ChainShapeConf.hpp.
◆ GetVertex()
|
inline |
Gets a vertex by index.
Definition at line 96 of file ChainShapeConf.hpp.
◆ GetNormal()
|
inline |
Gets the normal at the given index.
Definition at line 103 of file ChainShapeConf.hpp.
Friends And Related Function Documentation
◆ operator==
|
friend |
Equality operator.
Definition at line 110 of file ChainShapeConf.hpp.
◆ operator!=
|
friend |
Inequality operator.
Definition at line 119 of file ChainShapeConf.hpp.
Member Data Documentation
◆ vertexRadius
NonNegative<Length> playrho::d2::ChainShapeConf::vertexRadius = GetDefaultVertexRadius() |
Vertex radius.
This is the radius from the vertex that the shape's "skin" should extend outward by. While any edges — line segments between multiple vertices — are straight, corners between them (the vertices) are rounded and treated as rounded. Shapes with larger vertex radiuses compared to edge lengths therefore will be more prone to rolling or having other shapes more prone to roll off of them.
- Note
- This should be a non-negative value.
Definition at line 135 of file ChainShapeConf.hpp.
The documentation for this class was generated from the following files:
- Collision/Shapes/ChainShapeConf.hpp
- Collision/Shapes/ChainShapeConf.cpp