A collision response oriented description of the intersection of two convex shapes. More...
#include <Manifold.hpp>
Classes | |
struct | Conf |
Configuration data for manifold calculation. More... | |
struct | Point |
Data for a point of collision in a Manifold. More... | |
Public Types | |
enum | Type : std::uint8_t { e_unset, e_circles, e_faceA, e_faceB } |
using | size_type = std::remove_const< decltype(MaxManifoldPoints)>::type |
Size type. More... | |
using | CfIndex = ContactFeature::Index |
Contact feature index. More... | |
using | CfType = ContactFeature::Type |
Contact feature type. More... | |
Public Member Functions | |
Manifold ()=default | |
Manifold (const Manifold ©)=default | |
Copy constructor. More... | |
PLAYRHO_CONSTEXPR Type | GetType () const noexcept |
PLAYRHO_CONSTEXPR size_type | GetPointCount () const noexcept |
PLAYRHO_CONSTEXPR ContactFeature | GetContactFeature (size_type index) const noexcept |
Gets the contact feature for the given index. More... | |
PLAYRHO_CONSTEXPR Momentum2 | GetContactImpulses (size_type index) const noexcept |
Gets the contact impulses for the given index. More... | |
void | SetContactImpulses (size_type index, Momentum2 value) noexcept |
Sets the contact impulses for the given index. More... | |
const Point & | GetPoint (size_type index) const noexcept |
Gets the point identified by the given index. More... | |
void | SetPointImpulses (size_type index, Momentum n, Momentum t) |
Sets the point impulses for the given index. More... | |
void | AddPoint (const Point &mp) noexcept |
void | AddPoint (CfType type, CfIndex index, Length2 point) noexcept |
Adds a new point with the given data. More... | |
PLAYRHO_CONSTEXPR UnitVec | GetLocalNormal () const noexcept |
Gets the local normal for a face-type manifold. More... | |
PLAYRHO_CONSTEXPR Length2 | GetLocalPoint () const noexcept |
Gets the local point. More... | |
PLAYRHO_CONSTEXPR Length2 | GetOpposingPoint (size_type index) const noexcept |
Gets the opposing point. More... | |
Static Public Member Functions | |
static Manifold | GetForCircles (Length2 vA, CfIndex iA, Length2 vB, CfIndex iB) noexcept |
static Manifold | GetForFaceA (UnitVec normalA, Length2 faceA) noexcept |
static Manifold | GetForFaceA (UnitVec ln, Length2 lp, const Point &mp1) noexcept |
static Manifold | GetForFaceA (UnitVec ln, Length2 lp, const Point &mp1, const Point &mp2) noexcept |
static Manifold | GetForFaceB (UnitVec ln, Length2 lp) noexcept |
static Manifold | GetForFaceB (UnitVec ln, Length2 lp, const Point &mp1) noexcept |
static Manifold | GetForFaceB (UnitVec ln, Length2 lp, const Point &mp1, const Point &mp2) noexcept |
static Manifold | GetForFaceA (UnitVec na, CfIndex ia, Length2 pa) noexcept |
Gets the face A manifold for the given data. More... | |
static Manifold | GetForFaceB (UnitVec nb, CfIndex ib, Length2 pb) noexcept |
Gets the face B manifold for the given data. More... | |
static Manifold | GetForFaceA (UnitVec na, CfIndex ia, Length2 pa, CfType tb0, CfIndex ib0, Length2 pb0) noexcept |
Gets the face A manifold for the given data. More... | |
static Manifold | GetForFaceB (UnitVec nb, CfIndex ib, Length2 pb, CfType ta0, CfIndex ia0, Length2 pa0) noexcept |
Gets the face B manifold for the given data. More... | |
static Manifold | GetForFaceA (UnitVec na, CfIndex ia, Length2 pa, CfType tb0, CfIndex ib0, Length2 pb0, CfType tb1, CfIndex ib1, Length2 pb1) noexcept |
Gets the face A manifold for the given data. More... | |
static Manifold | GetForFaceB (UnitVec nb, CfIndex ib, Length2 pb, CfType ta0, CfIndex ia0, Length2 pa0, CfType ta1, CfIndex ia1, Length2 pa1) noexcept |
Gets the face B manifold for the given data. More... | |
Related Functions | |
(Note that these are not member functions.) | |
bool | operator== (const Manifold &lhs, const Manifold &rhs) noexcept |
Manifold equality operator. More... | |
bool | operator!= (const Manifold &lhs, const Manifold &rhs) noexcept |
Manifold inequality operator. More... | |
Manifold | CollideShapes (const DistanceProxy &shapeA, const Transformation &xfA, const DistanceProxy &shapeB, const Transformation &xfB, Manifold::Conf conf=GetDefaultManifoldConf()) |
Calculates the relevant collision manifold. More... | |
template<> | |
PLAYRHO_CONSTEXPR bool | IsValid (const d2::Manifold &value) noexcept |
Gets whether the given manifold is valid. More... | |
WorldManifold | GetWorldManifold (const Manifold &manifold, Transformation xfA, Length radiusA, Transformation xfB, Length radiusB) |
Gets the world manifold for the given data. More... | |
Detailed Description
A collision response oriented description of the intersection of two convex shapes.
This describes zero, one, or two points of contact for which impulses should be applied to most naturally resolve those contacts. Ideally the manifold is calculated at the earliest point in time of contact occurring. The further past that time, the less natural contact resolution of solid bodies will be - eventually resulting in oddities like tunneling.
Multiple types of contact are supported: clip point versus plane with radius, point versus point with radius (circles). Contacts are stored in this way so that position correction can account for movement, which is critical for continuous physics. All contact scenarios must be expressed in one of these types.
Conceptually, a manifold represents the intersection of two convex sets (which is itself a convex set) and a solution for moving the sets away from each other to eliminate the intersection.
- Note
- The local point and local normal usage depends on the manifold type. For details, see the documentation associated with the different manifold types.
- Every point adds computational overhead to the collision response calculation - so express collision manifolds with one point if possible instead of two.
- While this data structure is at least 58-bytes large (60-bytes on one 64-bit platform), it is intentionally 64-byte aligned making it 64-byte large (on 64-bit platforms with 4-byte Real).
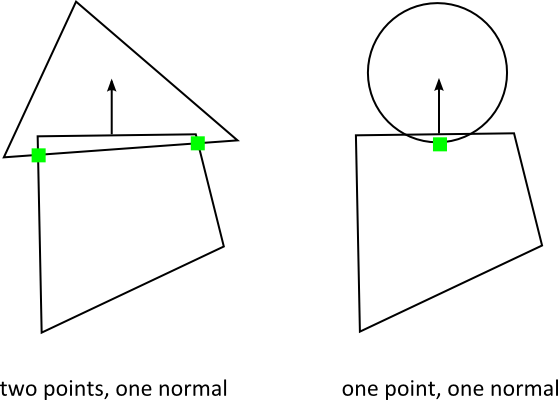
- See also
- Contact, PositionConstraint, VelocityConstraint
- https://en.wikipedia.org/wiki/Convex_set
- http://box2d.org/files/GDC2007/GDC2007_Catto_Erin_Physics2.ppt
- Examples
- World.cpp.
Definition at line 64 of file Manifold.hpp.
Member Typedef Documentation
◆ size_type
using playrho::d2::Manifold::size_type = std::remove_const<decltype(MaxManifoldPoints)>::type |
Size type.
Definition at line 69 of file Manifold.hpp.
◆ CfIndex
Contact feature index.
Definition at line 72 of file Manifold.hpp.
◆ CfType
Contact feature type.
Definition at line 75 of file Manifold.hpp.
Member Enumeration Documentation
◆ Type
enum playrho::d2::Manifold::Type : std::uint8_t |
Manifold type.
- Note
- This is by design a 1-byte sized type.
Enumerator | |
---|---|
e_unset | Unset type. Manifold is unset. For manifolds of this type: the point count is zero, point data is undefined, and all other properties are invalid. |
e_circles | Circles type. Manifold is for circle-to-circle like collisions.
|
e_faceA | Face-A type. Indicates: local point is center of face A, local normal is normal on shape A, and the local points of Point instances are the local center of circle B or a clip point of polygon B where the contact feature will be |
e_faceB | Face-B type. Indicates: local point is center of face B, local normal is normal on shape B, and the local points of Point instances are the local center of circle A or a clip point of polygon A where the contact feature will be |
Definition at line 81 of file Manifold.hpp.
Constructor & Destructor Documentation
◆ Manifold() [1/2]
|
default |
Default constructor.
Constructs an unset-type manifold. For an unset-type manifold: point count is zero, point data is undefined, and all other properties are invalid.
◆ Manifold() [2/2]
|
default |
Copy constructor.
Member Function Documentation
◆ GetForCircles()
|
inlinestaticnoexcept |
Gets a circles-typed manifold with one point.
- Parameters
-
vA Local center of "circle" A. iA Index of vertex from shape A representing the local center of "circle" A. vB Local center of "circle" B. iB Index of vertex from shape B representing the local center of "circle" B.
Definition at line 165 of file Manifold.hpp.
◆ GetForFaceA() [1/6]
|
inlinestaticnoexcept |
Gets a face A typed manifold.
- Parameters
-
normalA Local normal of the face from polygon A. faceA Any point in local coordinates on the face whose normal was provided.
Definition at line 177 of file Manifold.hpp.
◆ GetForFaceA() [2/6]
|
inlinestaticnoexcept |
Gets a face A typed manifold.
- Parameters
-
ln Normal on polygon A. lp Center of face A. mp1 Manifold point 1 (of 1).
Definition at line 186 of file Manifold.hpp.
◆ GetForFaceA() [3/6]
|
inlinestaticnoexcept |
Gets a face A typed manifold.
- Parameters
-
ln Normal on polygon A. lp Center of face A. mp1 Manifold point 1 (of 2). mp2 Manifold point 2 (of 2).
Definition at line 198 of file Manifold.hpp.
◆ GetForFaceB() [1/6]
Gets a face B typed manifold.
- Parameters
-
ln Normal on polygon B. lp Center of face B.
Definition at line 212 of file Manifold.hpp.
◆ GetForFaceB() [2/6]
|
inlinestaticnoexcept |
Gets a face B typed manifold.
- Parameters
-
ln Normal on polygon B. lp Center of face B. mp1 Manifold point 1.
Definition at line 221 of file Manifold.hpp.
◆ GetForFaceB() [3/6]
|
inlinestaticnoexcept |
Gets a face B typed manifold.
- Parameters
-
ln Normal on polygon B. lp Center of face B. mp1 Manifold point 1 (of 2). mp2 Manifold point 2 (of 2).
Definition at line 233 of file Manifold.hpp.
◆ GetForFaceA() [4/6]
|
inlinestaticnoexcept |
Gets the face A manifold for the given data.
Definition at line 243 of file Manifold.hpp.
◆ GetForFaceB() [4/6]
|
inlinestaticnoexcept |
Gets the face B manifold for the given data.
Definition at line 252 of file Manifold.hpp.
◆ GetForFaceA() [5/6]
|
inlinestaticnoexcept |
Gets the face A manifold for the given data.
Definition at line 261 of file Manifold.hpp.
◆ GetForFaceB() [5/6]
|
inlinestaticnoexcept |
Gets the face B manifold for the given data.
Definition at line 271 of file Manifold.hpp.
◆ GetForFaceA() [6/6]
|
inlinestaticnoexcept |
Gets the face A manifold for the given data.
Definition at line 281 of file Manifold.hpp.
◆ GetForFaceB() [6/6]
|
inlinestaticnoexcept |
Gets the face B manifold for the given data.
Definition at line 292 of file Manifold.hpp.
◆ GetType()
|
inlinenoexcept |
Gets the type of this manifold.
- Note
- This must be a constant expression in order to use it in the context of the
IsValid
specialized template function for it.
Definition at line 317 of file Manifold.hpp.
◆ GetPointCount()
|
inlinenoexcept |
Gets the manifold point count.
This is the count of contact points for this manifold. Only up to this many points can be validly accessed using the GetPoint()
method.
- Note
- Non-zero values indicate that the two shapes are touching.
- Returns
- Value between 0 and
MaxManifoldPoints
.
- See also
- MaxManifoldPoints.
- AddPoint().
- GetPoint().
Definition at line 332 of file Manifold.hpp.
◆ GetContactFeature()
|
inlinenoexcept |
Gets the contact feature for the given index.
Definition at line 335 of file Manifold.hpp.
◆ GetContactImpulses()
|
inlinenoexcept |
Gets the contact impulses for the given index.
- Returns
- Pair of impulses where the first impulse is the "normal impulse" and the second impulse is the "tangent impulse".
Definition at line 344 of file Manifold.hpp.
◆ SetContactImpulses()
Sets the contact impulses for the given index.
Sets the contact impulses for the given index where the first impulse is the "normal impulse" and the second impulse is the "tangent impulse".
Definition at line 353 of file Manifold.hpp.
◆ GetPoint()
Gets the point identified by the given index.
Definition at line 361 of file Manifold.hpp.
◆ SetPointImpulses()
Sets the point impulses for the given index.
Definition at line 368 of file Manifold.hpp.
◆ AddPoint() [1/2]
|
inlinenoexcept |
Adds a new point.
This can be called once for circle type manifolds, and up to twice for face-A or face-B type manifolds. GetPointCount()
can be called to find out how many points have already been added.
- Warning
- Behavior is undefined if this object's type is e_unset.
- Behavior is undefined if this is called more than twice.
Definition at line 495 of file Manifold.hpp.
◆ AddPoint() [2/2]
Adds a new point with the given data.
Definition at line 508 of file Manifold.hpp.
◆ GetLocalNormal()
|
inlinenoexcept |
Gets the local normal for a face-type manifold.
- Note
- Only valid for face-A or face-B type manifolds.
- Warning
- Behavior is undefined for unset (e_unset) type manifolds.
- Behavior is undefined for circles (e_circles) type manifolds.
- Returns
- Local normal if the manifold type is face A or face B, else invalid value.
Definition at line 391 of file Manifold.hpp.
◆ GetLocalPoint()
|
inlinenoexcept |
Gets the local point.
This is the: local center of "circle" A for circles-type manifolds; the center of face A for face-A-type manifolds; or the center of face B for face-B-type manifolds.
- Note
- Only valid for circle, face-A, or face-B type manifolds.
- Warning
- Behavior is undefined for unset (e_unset) type manifolds.
- Returns
- Local point.
Definition at line 406 of file Manifold.hpp.
◆ GetOpposingPoint()
|
inlinenoexcept |
Gets the opposing point.
Definition at line 413 of file Manifold.hpp.
Friends And Related Function Documentation
◆ operator==()
Manifold equality operator.
- Note
- In-so-far as manifold points are concerned, order doesn't matter; only whether the two manifolds have the same point set.
Definition at line 813 of file Manifold.cpp.
◆ operator!=()
Manifold inequality operator.
Determines whether the two given manifolds are not equal.
Definition at line 890 of file Manifold.cpp.
◆ CollideShapes()
|
related |
Calculates the relevant collision manifold.
- Note
- The returned touching state information typically agrees with that returned from the distance-proxy-based
TestOverlap
function. This is not always the case however especially when the separation or overlap distance is closer to zero.
Definition at line 388 of file Manifold.cpp.
◆ IsValid()
|
related |
Gets whether the given manifold is valid.
Definition at line 620 of file Manifold.hpp.
◆ GetWorldManifold()
|
related |
Gets the world manifold for the given data.
- Precondition
- The given manifold input has between 0 and 2 points.
- Parameters
-
manifold Manifold to use. Uses the manifold's type, local point, local normal, point-count, and the indexed-points' local point data. xfA Transformation A. radiusA Radius of shape A. xfB Transformation B. radiusB Radius of shape B.
- Returns
- World manifold value for the given inputs which will have the same number of points as the given manifold has. The returned world manifold points will be the mid-points of the manifold intersection.
Definition at line 105 of file WorldManifold.cpp.
The documentation for this class was generated from the following files:
- Collision/Manifold.hpp
- Collision/WorldManifold.hpp