Polygon shape configuration. More...
#include <PolygonShapeConf.hpp>
Public Member Functions | |
PolygonShapeConf () | |
PolygonShapeConf (Length hx, Length hy, const PolygonShapeConf &conf=GetDefaultConf()) noexcept | |
Initializing constructor for a 4-sided box polygon. More... | |
PolygonShapeConf (Span< const Length2 > points, const PolygonShapeConf &conf=GetDefaultConf()) noexcept | |
Creates a convex hull from the given array of local points. More... | |
PolygonShapeConf & | UseVertexRadius (NonNegative< Length > value) noexcept |
Uses the given vertex radius. More... | |
PolygonShapeConf & | UseVertices (const std::vector< Length2 > &verts) noexcept |
Uses the given vertices. More... | |
PolygonShapeConf & | SetAsBox (Length hx, Length hy) noexcept |
Sets the vertices to represent an axis-aligned box centered on the local origin. More... | |
PolygonShapeConf & | SetAsBox (Length hx, Length hy, Length2 center, Angle angle) noexcept |
Sets the vertices for the described box. More... | |
PolygonShapeConf & | Set (Span< const Length2 > verts) noexcept |
Sets the vertices to a convex hull of the given ones. More... | |
PolygonShapeConf & | Set (const VertexSet &points) noexcept |
Sets the vertices to a convex hull of the given ones. More... | |
PolygonShapeConf & | Transform (Transformation xfm) noexcept |
Transforms the vertices by the given transformation. More... | |
PolygonShapeConf & | Transform (const Mat22 &m) noexcept |
Transforms the vertices by the given transformation matrix. More... | |
VertexCounter | GetVertexCount () const noexcept |
Length2 | GetVertex (VertexCounter index) const |
UnitVec | GetNormal (VertexCounter index) const |
Span< const Length2 > | GetVertices () const noexcept |
Span< const UnitVec > | GetNormals () const noexcept |
Gets the span of normals. More... | |
Length2 | GetCentroid () const noexcept |
Gets the centroid. More... | |
![]() | |
PLAYRHO_CONSTEXPR | ShapeBuilder ()=default |
Default constructor. More... | |
PLAYRHO_CONSTEXPR | ShapeBuilder (const BaseShapeConf &value) noexcept |
Initializing constructor. More... | |
PLAYRHO_CONSTEXPR PolygonShapeConf & | UseFriction (NonNegative< Real > value) noexcept |
Uses the given friction. More... | |
PLAYRHO_CONSTEXPR PolygonShapeConf & | UseRestitution (Finite< Real > value) noexcept |
Uses the given restitution. More... | |
PLAYRHO_CONSTEXPR PolygonShapeConf & | UseDensity (NonNegative< AreaDensity > value) noexcept |
Uses the given density. More... | |
Static Public Member Functions | |
static PLAYRHO_CONSTEXPR NonNegative< Length > | GetDefaultVertexRadius () noexcept |
Gets the default vertex radius for the PolygonShapeConf . More... | |
static PolygonShapeConf | GetDefaultConf () noexcept |
Gets the default configuration for a PolygonShapeConf . More... | |
Public Attributes | |
NonNegative< Length > | vertexRadius = GetDefaultVertexRadius() |
Vertex radius. More... | |
![]() | |
NonNegative< Real > | friction = NonNegative<Real>{Real{2} / Real{10}} |
Friction coefficient. More... | |
Finite< Real > | restitution = Finite<Real>{0} |
Restitution (elasticity) of the associated shape. More... | |
NonNegative< AreaDensity > | density = NonNegative<AreaDensity>{0_kgpm2} |
Area density of the associated shape. More... | |
Friends | |
bool | operator== (const PolygonShapeConf &lhs, const PolygonShapeConf &rhs) noexcept |
Equality operator. More... | |
bool | operator!= (const PolygonShapeConf &lhs, const PolygonShapeConf &rhs) noexcept |
Inequality operator. More... | |
Related Functions | |
(Note that these are not member functions.) | |
Length2 | GetEdge (const PolygonShapeConf &shape, VertexCounter index) |
Detailed Description
Polygon shape configuration.
A convex polygon. The interior of the polygon is to the left of each edge. Polygons maximum number of vertices is defined by MaxShapeVertices
. In most cases you should not need many vertices for a convex polygon.
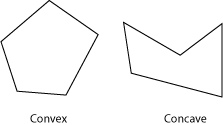
- Note
- This data structure is 64-bytes large (with 4-byte Real).
- Examples
- HelloWorld.cpp, and World.cpp.
Definition at line 41 of file PolygonShapeConf.hpp.
Constructor & Destructor Documentation
◆ PolygonShapeConf() [1/3]
|
default |
◆ PolygonShapeConf() [2/3]
|
noexcept |
Initializing constructor for a 4-sided box polygon.
Definition at line 28 of file PolygonShapeConf.cpp.
◆ PolygonShapeConf() [3/3]
|
explicitnoexcept |
Creates a convex hull from the given array of local points.
- Note
- The size of the span must be in the range [1,
MaxShapeVertices
].
- Warning
- the points may be re-ordered, even if they form a convex polygon
- collinear points are handled but not removed. Collinear points may lead to poor stacking behavior.
Definition at line 35 of file PolygonShapeConf.cpp.
Member Function Documentation
◆ GetDefaultVertexRadius()
|
inlinestaticnoexcept |
Gets the default vertex radius for the PolygonShapeConf
.
Definition at line 45 of file PolygonShapeConf.hpp.
◆ GetDefaultConf()
|
inlinestaticnoexcept |
Gets the default configuration for a PolygonShapeConf
.
Definition at line 51 of file PolygonShapeConf.hpp.
◆ UseVertexRadius()
|
inlinenoexcept |
Uses the given vertex radius.
- Examples
- World.cpp.
Definition at line 191 of file PolygonShapeConf.hpp.
◆ UseVertices()
|
noexcept |
Uses the given vertices.
Definition at line 69 of file PolygonShapeConf.cpp.
◆ SetAsBox() [1/2]
|
noexcept |
Sets the vertices to represent an axis-aligned box centered on the local origin.
- Parameters
-
hx the half-width. hy the half-height.
- Examples
- HelloWorld.cpp, and World.cpp.
Definition at line 42 of file PolygonShapeConf.cpp.
◆ SetAsBox() [2/2]
|
noexcept |
Sets the vertices for the described box.
Definition at line 74 of file PolygonShapeConf.cpp.
◆ Set() [1/2]
|
noexcept |
Sets the vertices to a convex hull of the given ones.
- Note
- The size of the span must be in the range [1,
MaxShapeVertices
].
- Warning
- Points may be re-ordered, even if they form a convex polygon
- Collinear points are handled but not removed. Collinear points may lead to poor stacking behavior.
Definition at line 104 of file PolygonShapeConf.cpp.
◆ Set() [2/2]
|
noexcept |
Sets the vertices to a convex hull of the given ones.
- Note
- The size of the span must be in the range [1,
MaxShapeVertices
].
- Warning
- Points may be re-ordered, even if they form a convex polygon
- Collinear points are handled but not removed. Collinear points may lead to poor stacking behavior.
Definition at line 115 of file PolygonShapeConf.cpp.
◆ Transform() [1/2]
|
noexcept |
Transforms the vertices by the given transformation.
Definition at line 82 of file PolygonShapeConf.cpp.
◆ Transform() [2/2]
|
noexcept |
Transforms the vertices by the given transformation matrix.
Definition at line 93 of file PolygonShapeConf.cpp.
◆ GetVertexCount()
|
inlinenoexcept |
Gets the vertex count.
- Returns
- value between 0 and
MaxShapeVertices
inclusive.
- See also
- MaxShapeVertices
Definition at line 123 of file PolygonShapeConf.hpp.
◆ GetVertex()
|
inline |
Gets a vertex by index.
Vertices go counter-clockwise.
Definition at line 130 of file PolygonShapeConf.hpp.
◆ GetNormal()
|
inline |
Gets a normal by index.
These are 90-degree clockwise-rotated (outward-facing) unit-vectors of the edges defined by consecutive pairs of vertices starting with vertex 0.
- Parameters
-
index Index of the normal to get.
- Returns
- Normal for the given index.
Definition at line 142 of file PolygonShapeConf.hpp.
◆ GetVertices()
Gets the span of vertices.
Vertices go counter-clockwise.
Definition at line 150 of file PolygonShapeConf.hpp.
◆ GetNormals()
Gets the span of normals.
Definition at line 156 of file PolygonShapeConf.hpp.
◆ GetCentroid()
|
inlinenoexcept |
Gets the centroid.
Definition at line 162 of file PolygonShapeConf.hpp.
Friends And Related Function Documentation
◆ operator==
|
friend |
Equality operator.
Definition at line 106 of file PolygonShapeConf.hpp.
◆ operator!=
|
friend |
Inequality operator.
Definition at line 115 of file PolygonShapeConf.hpp.
◆ GetEdge()
|
related |
Gets the identified edge of the given polygon shape.
- Note
- This must not be called for shapes with less than 2 vertices.
- Warning
- Behavior is undefined if called for a shape with less than 2 vertices.
Definition at line 157 of file PolygonShapeConf.cpp.
Member Data Documentation
◆ vertexRadius
NonNegative<Length> playrho::d2::PolygonShapeConf::vertexRadius = GetDefaultVertexRadius() |
Vertex radius.
This is the radius from the vertex that the shape's "skin" should extend outward by. While any edges — line segments between multiple vertices — are straight, corners between them (the vertices) are rounded and treated as rounded. Shapes with larger vertex radiuses compared to edge lengths therefore will be more prone to rolling or having other shapes more prone to roll off of them.
- Note
- This should be a non-negative value.
Definition at line 175 of file PolygonShapeConf.hpp.
The documentation for this class was generated from the following files:
- Collision/Shapes/PolygonShapeConf.hpp
- Collision/Shapes/PolygonShapeConf.cpp