#include <DistanceJoint.hpp>
Public Member Functions | |
DistanceJoint (const DistanceJointConf &data) | |
Initializing constructor. More... | |
void | Accept (JointVisitor &visitor) const override |
Accepts a visitor. More... | |
void | Accept (JointVisitor &visitor) override |
Accepts a visitor. More... | |
Length2 | GetAnchorA () const override |
Get the anchor point on body-A in world coordinates. More... | |
Length2 | GetAnchorB () const override |
Get the anchor point on body-B in world coordinates. More... | |
Momentum2 | GetLinearReaction () const override |
Get the linear reaction on body-B at the joint anchor. More... | |
AngularMomentum | GetAngularReaction () const override |
Get the angular reaction on body-B. More... | |
Length2 | GetLocalAnchorA () const noexcept |
Gets the local anchor point relative to body A's origin. More... | |
Length2 | GetLocalAnchorB () const noexcept |
Gets the local anchor point relative to body B's origin. More... | |
void | SetLength (Length length) noexcept |
Sets the natural length. More... | |
Length | GetLength () const noexcept |
Gets the length. More... | |
void | SetFrequency (NonNegative< Frequency > frequency) noexcept |
Sets frequency. More... | |
NonNegative< Frequency > | GetFrequency () const noexcept |
Gets the frequency. More... | |
void | SetDampingRatio (Real ratio) noexcept |
Sets the damping ratio. More... | |
Real | GetDampingRatio () const noexcept |
Gets damping ratio. More... | |
![]() | |
virtual | ~Joint () noexcept=default |
Body * | GetBodyA () const noexcept |
Gets the first body attached to this joint. More... | |
Body * | GetBodyB () const noexcept |
Gets the second body attached to this joint. More... | |
void * | GetUserData () const noexcept |
Get the user data pointer. More... | |
void | SetUserData (void *data) noexcept |
Set the user data pointer. More... | |
bool | GetCollideConnected () const noexcept |
Gets collide connected. More... | |
virtual bool | ShiftOrigin (const Length2) |
Shifts the origin for any points stored in world coordinates. More... | |
Static Public Member Functions | |
static bool | IsOkay (const DistanceJointConf &data) noexcept |
Is the given definition okay. More... | |
![]() | |
static bool | IsOkay (const JointConf &def) noexcept |
Is the given definition okay. More... | |
Related Functions | |
(Note that these are not member functions.) | |
DistanceJointConf | GetDistanceJointConf (const DistanceJoint &joint) noexcept |
Gets the definition data for the given joint. More... | |
![]() | |
bool | IsEnabled (const Joint &j) noexcept |
Short-cut function to determine if both bodies are enabled. More... | |
void | SetAwake (Joint &j) noexcept |
Wakes up the joined bodies. More... | |
JointCounter | GetWorldIndex (const Joint *joint) |
Gets the world index of the given joint. More... | |
JointType | GetType (const Joint &joint) noexcept |
Gets the type of the given joint. More... | |
Additional Inherited Members | |
![]() | |
enum | LimitState { e_inactiveLimit, e_atLowerLimit, e_atUpperLimit, e_equalLimits } |
Limit state. More... | |
![]() | |
Joint (const JointConf &def) | |
Initializing constructor. More... | |
Detailed Description
Distance Joint.
A distance joint constrains two points on two bodies to remain at a fixed distance from each other. You can view this as a massless, rigid rod.
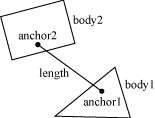
Definition at line 40 of file DistanceJoint.hpp.
Constructor & Destructor Documentation
◆ DistanceJoint()
playrho::d2::DistanceJoint::DistanceJoint | ( | const DistanceJointConf & | data | ) |
Initializing constructor.
- Attention
- To create or use the joint within a world instance, call that world instance's create joint method instead of calling this constructor directly.
- See also
- World::CreateJoint
Definition at line 58 of file DistanceJoint.cpp.
Member Function Documentation
◆ IsOkay()
|
staticnoexcept |
Is the given definition okay.
Definition at line 49 of file DistanceJoint.cpp.
◆ Accept() [1/2]
|
overridevirtual |
Accepts a visitor.
This is the Accept method definition of a "visitor design pattern" for for doing joint subclass specific types of processing for a constant joint.
Implements playrho::d2::Joint.
Definition at line 69 of file DistanceJoint.cpp.
◆ Accept() [2/2]
|
overridevirtual |
Accepts a visitor.
This is the Accept method definition of a "visitor design pattern" for for doing joint subclass specific types of processing.
Implements playrho::d2::Joint.
Definition at line 74 of file DistanceJoint.cpp.
◆ GetAnchorA()
|
overridevirtual |
Get the anchor point on body-A in world coordinates.
Implements playrho::d2::Joint.
Definition at line 249 of file DistanceJoint.cpp.
◆ GetAnchorB()
|
overridevirtual |
Get the anchor point on body-B in world coordinates.
Implements playrho::d2::Joint.
Definition at line 254 of file DistanceJoint.cpp.
◆ GetLinearReaction()
|
overridevirtual |
Get the linear reaction on body-B at the joint anchor.
Implements playrho::d2::Joint.
Definition at line 259 of file DistanceJoint.cpp.
◆ GetAngularReaction()
|
overridevirtual |
Get the angular reaction on body-B.
Implements playrho::d2::Joint.
Definition at line 264 of file DistanceJoint.cpp.
◆ GetLocalAnchorA()
|
inlinenoexcept |
Gets the local anchor point relative to body A's origin.
Definition at line 61 of file DistanceJoint.hpp.
◆ GetLocalAnchorB()
|
inlinenoexcept |
Gets the local anchor point relative to body B's origin.
Definition at line 64 of file DistanceJoint.hpp.
◆ SetLength()
|
inlinenoexcept |
Sets the natural length.
- Note
- Manipulating the length can lead to non-physical behavior when the frequency is zero.
Definition at line 111 of file DistanceJoint.hpp.
◆ GetLength()
|
inlinenoexcept |
Gets the length.
Definition at line 116 of file DistanceJoint.hpp.
◆ SetFrequency()
|
inlinenoexcept |
Sets frequency.
Definition at line 121 of file DistanceJoint.hpp.
◆ GetFrequency()
|
inlinenoexcept |
Gets the frequency.
Definition at line 126 of file DistanceJoint.hpp.
◆ SetDampingRatio()
|
inlinenoexcept |
Sets the damping ratio.
Definition at line 131 of file DistanceJoint.hpp.
◆ GetDampingRatio()
|
inlinenoexcept |
Gets damping ratio.
Definition at line 136 of file DistanceJoint.hpp.
Friends And Related Function Documentation
◆ GetDistanceJointConf()
|
related |
Gets the definition data for the given joint.
Definition at line 39 of file DistanceJointConf.cpp.
The documentation for this class was generated from the following files:
- Dynamics/Joints/DistanceJoint.hpp
- Dynamics/Joints/DistanceJoint.cpp
- Dynamics/Joints/DistanceJointConf.hpp