#include <PrismaticJoint.hpp>
Public Member Functions | |
PrismaticJoint (const PrismaticJointConf &def) | |
Initializing constructor. More... | |
void | Accept (JointVisitor &visitor) const override |
Accepts a visitor. More... | |
void | Accept (JointVisitor &visitor) override |
Accepts a visitor. More... | |
Length2 | GetAnchorA () const override |
Get the anchor point on body-A in world coordinates. More... | |
Length2 | GetAnchorB () const override |
Get the anchor point on body-B in world coordinates. More... | |
Momentum2 | GetLinearReaction () const override |
Get the linear reaction on body-B at the joint anchor. More... | |
AngularMomentum | GetAngularReaction () const override |
Get the angular reaction on body-B. More... | |
Length2 | GetLocalAnchorA () const |
Gets the local anchor point relative to body A's origin. More... | |
Length2 | GetLocalAnchorB () const |
Gets the local anchor point relative to body B's origin. More... | |
UnitVec | GetLocalAxisA () const |
Gets local joint axis relative to body-A. More... | |
Angle | GetReferenceAngle () const |
Gets the reference angle. More... | |
bool | IsLimitEnabled () const noexcept |
Is the joint limit enabled? More... | |
void | EnableLimit (bool flag) noexcept |
Enable/disable the joint limit. More... | |
Length | GetLowerLimit () const noexcept |
Gets the lower joint limit. More... | |
Length | GetUpperLimit () const noexcept |
Gets the upper joint limit. More... | |
void | SetLimits (Length lower, Length upper) noexcept |
Sets the joint limits. More... | |
bool | IsMotorEnabled () const noexcept |
Is the joint motor enabled? More... | |
void | EnableMotor (bool flag) noexcept |
Enable/disable the joint motor. More... | |
void | SetMotorSpeed (AngularVelocity speed) noexcept |
Sets the motor speed. More... | |
AngularVelocity | GetMotorSpeed () const noexcept |
Gets the motor speed. More... | |
void | SetMaxMotorForce (Force force) noexcept |
Sets the maximum motor force. More... | |
Force | GetMaxMotorForce () const noexcept |
Gets the maximum motor force. More... | |
Momentum | GetMotorImpulse () const noexcept |
Gets the current motor impulse. More... | |
LimitState | GetLimitState () const noexcept |
Gets the current limit state. More... | |
![]() | |
virtual | ~Joint () noexcept=default |
Body * | GetBodyA () const noexcept |
Gets the first body attached to this joint. More... | |
Body * | GetBodyB () const noexcept |
Gets the second body attached to this joint. More... | |
void * | GetUserData () const noexcept |
Get the user data pointer. More... | |
void | SetUserData (void *data) noexcept |
Set the user data pointer. More... | |
bool | GetCollideConnected () const noexcept |
Gets collide connected. More... | |
virtual bool | ShiftOrigin (const Length2) |
Shifts the origin for any points stored in world coordinates. More... | |
Related Functions | |
(Note that these are not member functions.) | |
Length | GetJointTranslation (const PrismaticJoint &joint) noexcept |
Get the current joint translation. More... | |
LinearVelocity | GetLinearVelocity (const PrismaticJoint &joint) noexcept |
Get the current joint translation speed. More... | |
Force | GetMotorForce (const PrismaticJoint &joint, Frequency inv_dt) noexcept |
Gets the current motor force for the given joint, given the inverse time step. More... | |
PrismaticJointConf | GetPrismaticJointConf (const PrismaticJoint &joint) noexcept |
Gets the definition data for the given joint. More... | |
![]() | |
bool | IsEnabled (const Joint &j) noexcept |
Short-cut function to determine if both bodies are enabled. More... | |
void | SetAwake (Joint &j) noexcept |
Wakes up the joined bodies. More... | |
JointCounter | GetWorldIndex (const Joint *joint) |
Gets the world index of the given joint. More... | |
JointType | GetType (const Joint &joint) noexcept |
Gets the type of the given joint. More... | |
Additional Inherited Members | |
![]() | |
enum | LimitState { e_inactiveLimit, e_atLowerLimit, e_atUpperLimit, e_equalLimits } |
Limit state. More... | |
![]() | |
static bool | IsOkay (const JointConf &def) noexcept |
Is the given definition okay. More... | |
![]() | |
Joint (const JointConf &def) | |
Initializing constructor. More... | |
Detailed Description
Prismatic Joint.
This joint provides one degree of freedom: translation along an axis fixed in body-A. Relative rotation is prevented.
- Note
- You can use a joint limit to restrict the range of motion and a joint motor to drive the motion or to model joint friction.
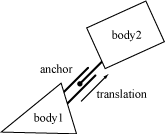
Definition at line 46 of file PrismaticJoint.hpp.
Constructor & Destructor Documentation
◆ PrismaticJoint()
playrho::d2::PrismaticJoint::PrismaticJoint | ( | const PrismaticJointConf & | def | ) |
Initializing constructor.
- Attention
- To create or use the joint within a world instance, call that world instance's create joint method instead of calling this constructor directly.
- See also
- World::CreateJoint
Definition at line 100 of file PrismaticJoint.cpp.
Member Function Documentation
◆ Accept() [1/2]
|
overridevirtual |
Accepts a visitor.
This is the Accept method definition of a "visitor design pattern" for for doing joint subclass specific types of processing for a constant joint.
Implements playrho::d2::Joint.
Definition at line 117 of file PrismaticJoint.cpp.
◆ Accept() [2/2]
|
overridevirtual |
Accepts a visitor.
This is the Accept method definition of a "visitor design pattern" for for doing joint subclass specific types of processing.
Implements playrho::d2::Joint.
Definition at line 122 of file PrismaticJoint.cpp.
◆ GetAnchorA()
|
overridevirtual |
Get the anchor point on body-A in world coordinates.
Implements playrho::d2::Joint.
Definition at line 524 of file PrismaticJoint.cpp.
◆ GetAnchorB()
|
overridevirtual |
Get the anchor point on body-B in world coordinates.
Implements playrho::d2::Joint.
Definition at line 529 of file PrismaticJoint.cpp.
◆ GetLinearReaction()
|
overridevirtual |
Get the linear reaction on body-B at the joint anchor.
Implements playrho::d2::Joint.
Definition at line 534 of file PrismaticJoint.cpp.
◆ GetAngularReaction()
|
overridevirtual |
Get the angular reaction on body-B.
Implements playrho::d2::Joint.
Definition at line 541 of file PrismaticJoint.cpp.
◆ GetLocalAnchorA()
|
inline |
Gets the local anchor point relative to body A's origin.
Definition at line 66 of file PrismaticJoint.hpp.
◆ GetLocalAnchorB()
|
inline |
Gets the local anchor point relative to body B's origin.
Definition at line 69 of file PrismaticJoint.hpp.
◆ GetLocalAxisA()
|
inline |
Gets local joint axis relative to body-A.
Definition at line 72 of file PrismaticJoint.hpp.
◆ GetReferenceAngle()
|
inline |
Gets the reference angle.
Definition at line 75 of file PrismaticJoint.hpp.
◆ IsLimitEnabled()
|
inlinenoexcept |
Is the joint limit enabled?
Definition at line 162 of file PrismaticJoint.hpp.
◆ EnableLimit()
|
noexcept |
Enable/disable the joint limit.
Definition at line 546 of file PrismaticJoint.cpp.
◆ GetLowerLimit()
|
inlinenoexcept |
Gets the lower joint limit.
Definition at line 152 of file PrismaticJoint.hpp.
◆ GetUpperLimit()
|
inlinenoexcept |
Gets the upper joint limit.
Definition at line 157 of file PrismaticJoint.hpp.
◆ SetLimits()
Sets the joint limits.
Definition at line 558 of file PrismaticJoint.cpp.
◆ IsMotorEnabled()
|
inlinenoexcept |
Is the joint motor enabled?
Definition at line 167 of file PrismaticJoint.hpp.
◆ EnableMotor()
|
noexcept |
Enable/disable the joint motor.
Definition at line 572 of file PrismaticJoint.cpp.
◆ SetMotorSpeed()
|
noexcept |
Sets the motor speed.
Definition at line 584 of file PrismaticJoint.cpp.
◆ GetMotorSpeed()
|
inlinenoexcept |
Gets the motor speed.
Definition at line 172 of file PrismaticJoint.hpp.
◆ SetMaxMotorForce()
|
noexcept |
Sets the maximum motor force.
Definition at line 596 of file PrismaticJoint.cpp.
◆ GetMaxMotorForce()
|
inlinenoexcept |
Gets the maximum motor force.
Definition at line 108 of file PrismaticJoint.hpp.
◆ GetMotorImpulse()
|
inlinenoexcept |
Gets the current motor impulse.
Definition at line 111 of file PrismaticJoint.hpp.
◆ GetLimitState()
|
inlinenoexcept |
Gets the current limit state.
- Note
- This will be
e_inactiveLimit
unless the joint limit has been enabled.
Definition at line 177 of file PrismaticJoint.hpp.
Friends And Related Function Documentation
◆ GetJointTranslation()
|
related |
Get the current joint translation.
Definition at line 608 of file PrismaticJoint.cpp.
◆ GetLinearVelocity()
|
related |
Get the current joint translation speed.
Definition at line 615 of file PrismaticJoint.cpp.
◆ GetMotorForce()
|
related |
Gets the current motor force for the given joint, given the inverse time step.
Definition at line 192 of file PrismaticJoint.hpp.
◆ GetPrismaticJointConf()
|
related |
Gets the definition data for the given joint.
Definition at line 40 of file PrismaticJointConf.cpp.
The documentation for this class was generated from the following files:
- Dynamics/Joints/PrismaticJoint.hpp
- Dynamics/Joints/PrismaticJoint.cpp
- Dynamics/Joints/PrismaticJointConf.hpp