#include <RevoluteJoint.hpp>
Public Member Functions | |
RevoluteJoint (const RevoluteJointConf &def) | |
Initializing constructor. More... | |
void | Accept (JointVisitor &visitor) const override |
Accepts a visitor. More... | |
void | Accept (JointVisitor &visitor) override |
Accepts a visitor. More... | |
Length2 | GetAnchorA () const override |
Get the anchor point on body-A in world coordinates. More... | |
Length2 | GetAnchorB () const override |
Get the anchor point on body-B in world coordinates. More... | |
Length2 | GetLocalAnchorA () const noexcept |
The local anchor point relative to body A's origin. More... | |
Length2 | GetLocalAnchorB () const noexcept |
The local anchor point relative to body B's origin. More... | |
Angle | GetReferenceAngle () const noexcept |
Get the reference angle. More... | |
bool | IsLimitEnabled () const noexcept |
Is the joint limit enabled? More... | |
void | EnableLimit (bool flag) |
Enable/disable the joint limit. More... | |
Angle | GetLowerLimit () const noexcept |
Get the lower joint limit. More... | |
Angle | GetUpperLimit () const noexcept |
Get the upper joint limit. More... | |
void | SetLimits (Angle lower, Angle upper) |
Set the joint limits. More... | |
bool | IsMotorEnabled () const noexcept |
Is the joint motor enabled? More... | |
void | EnableMotor (bool flag) |
Enable/disable the joint motor. More... | |
void | SetMotorSpeed (AngularVelocity speed) |
Set the angular motor speed. More... | |
AngularVelocity | GetMotorSpeed () const noexcept |
Gets the angular motor speed. More... | |
void | SetMaxMotorTorque (Torque torque) |
Set the maximum motor torque. More... | |
Torque | GetMaxMotorTorque () const noexcept |
Gets the max motor torque. More... | |
Momentum2 | GetLinearReaction () const override |
Get the linear reaction. More... | |
AngularMomentum | GetAngularReaction () const override |
Get the angular reaction due to the joint limit. More... | |
AngularMomentum | GetMotorImpulse () const noexcept |
Gets the current motor impulse. More... | |
LimitState | GetLimitState () const noexcept |
Gets the current limit state. More... | |
![]() | |
virtual | ~Joint () noexcept=default |
Body * | GetBodyA () const noexcept |
Gets the first body attached to this joint. More... | |
Body * | GetBodyB () const noexcept |
Gets the second body attached to this joint. More... | |
void * | GetUserData () const noexcept |
Get the user data pointer. More... | |
void | SetUserData (void *data) noexcept |
Set the user data pointer. More... | |
bool | GetCollideConnected () const noexcept |
Gets collide connected. More... | |
virtual bool | ShiftOrigin (const Length2) |
Shifts the origin for any points stored in world coordinates. More... | |
Related Functions | |
(Note that these are not member functions.) | |
Angle | GetJointAngle (const RevoluteJoint &joint) |
Gets the current joint angle. More... | |
AngularVelocity | GetAngularVelocity (const RevoluteJoint &joint) |
Gets the current joint angle speed. More... | |
Torque | GetMotorTorque (const RevoluteJoint &joint, Frequency inv_dt) noexcept |
Gets the current motor torque for the given joint given the inverse time step. More... | |
RevoluteJointConf | GetRevoluteJointConf (const RevoluteJoint &joint) noexcept |
Gets the definition data for the given joint. More... | |
![]() | |
bool | IsEnabled (const Joint &j) noexcept |
Short-cut function to determine if both bodies are enabled. More... | |
void | SetAwake (Joint &j) noexcept |
Wakes up the joined bodies. More... | |
JointCounter | GetWorldIndex (const Joint *joint) |
Gets the world index of the given joint. More... | |
JointType | GetType (const Joint &joint) noexcept |
Gets the type of the given joint. More... | |
Additional Inherited Members | |
![]() | |
enum | LimitState { e_inactiveLimit, e_atLowerLimit, e_atUpperLimit, e_equalLimits } |
Limit state. More... | |
![]() | |
static bool | IsOkay (const JointConf &def) noexcept |
Is the given definition okay. More... | |
![]() | |
Joint (const JointConf &def) | |
Initializing constructor. More... | |
Detailed Description
Revolute Joint.
A revolute joint constrains two bodies to share a common point while they are free to rotate about the point. The relative rotation about the shared point is the joint angle.
- Note
- You can limit the relative rotation with a joint limit that specifies a lower and upper angle. You can use a motor to drive the relative rotation about the shared point. A maximum motor torque is provided so that infinite forces are not generated.
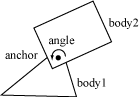
Definition at line 48 of file RevoluteJoint.hpp.
Constructor & Destructor Documentation
◆ RevoluteJoint()
playrho::d2::RevoluteJoint::RevoluteJoint | ( | const RevoluteJointConf & | def | ) |
Initializing constructor.
- Attention
- To create or use the joint within a world instance, call that world instance's create joint method instead of calling this constructor directly.
- See also
- World::CreateJoint
Definition at line 90 of file RevoluteJoint.cpp.
Member Function Documentation
◆ Accept() [1/2]
|
overridevirtual |
Accepts a visitor.
This is the Accept method definition of a "visitor design pattern" for for doing joint subclass specific types of processing for a constant joint.
Implements playrho::d2::Joint.
Definition at line 105 of file RevoluteJoint.cpp.
◆ Accept() [2/2]
|
overridevirtual |
Accepts a visitor.
This is the Accept method definition of a "visitor design pattern" for for doing joint subclass specific types of processing.
Implements playrho::d2::Joint.
Definition at line 110 of file RevoluteJoint.cpp.
◆ GetAnchorA()
|
overridevirtual |
Get the anchor point on body-A in world coordinates.
Implements playrho::d2::Joint.
Definition at line 453 of file RevoluteJoint.cpp.
◆ GetAnchorB()
|
overridevirtual |
Get the anchor point on body-B in world coordinates.
Implements playrho::d2::Joint.
Definition at line 458 of file RevoluteJoint.cpp.
◆ GetLocalAnchorA()
|
inlinenoexcept |
The local anchor point relative to body A's origin.
Definition at line 65 of file RevoluteJoint.hpp.
◆ GetLocalAnchorB()
|
inlinenoexcept |
The local anchor point relative to body B's origin.
Definition at line 68 of file RevoluteJoint.hpp.
◆ GetReferenceAngle()
|
inlinenoexcept |
Get the reference angle.
Definition at line 71 of file RevoluteJoint.hpp.
◆ IsLimitEnabled()
|
inlinenoexcept |
Is the joint limit enabled?
Definition at line 162 of file RevoluteJoint.hpp.
◆ EnableLimit()
void playrho::d2::RevoluteJoint::EnableLimit | ( | bool | flag | ) |
Enable/disable the joint limit.
Definition at line 510 of file RevoluteJoint.cpp.
◆ GetLowerLimit()
|
inlinenoexcept |
Get the lower joint limit.
Definition at line 167 of file RevoluteJoint.hpp.
◆ GetUpperLimit()
|
inlinenoexcept |
Get the upper joint limit.
Definition at line 172 of file RevoluteJoint.hpp.
◆ SetLimits()
Set the joint limits.
Definition at line 522 of file RevoluteJoint.cpp.
◆ IsMotorEnabled()
|
inlinenoexcept |
Is the joint motor enabled?
Definition at line 177 of file RevoluteJoint.hpp.
◆ EnableMotor()
void playrho::d2::RevoluteJoint::EnableMotor | ( | bool | flag | ) |
Enable/disable the joint motor.
Definition at line 474 of file RevoluteJoint.cpp.
◆ SetMotorSpeed()
void playrho::d2::RevoluteJoint::SetMotorSpeed | ( | AngularVelocity | speed | ) |
Set the angular motor speed.
Definition at line 486 of file RevoluteJoint.cpp.
◆ GetMotorSpeed()
|
inlinenoexcept |
Gets the angular motor speed.
Definition at line 182 of file RevoluteJoint.hpp.
◆ SetMaxMotorTorque()
void playrho::d2::RevoluteJoint::SetMaxMotorTorque | ( | Torque | torque | ) |
Set the maximum motor torque.
Definition at line 498 of file RevoluteJoint.cpp.
◆ GetMaxMotorTorque()
|
inlinenoexcept |
Gets the max motor torque.
Definition at line 187 of file RevoluteJoint.hpp.
◆ GetLinearReaction()
|
overridevirtual |
Get the linear reaction.
Implements playrho::d2::Joint.
Definition at line 463 of file RevoluteJoint.cpp.
◆ GetAngularReaction()
|
overridevirtual |
Get the angular reaction due to the joint limit.
Implements playrho::d2::Joint.
Definition at line 468 of file RevoluteJoint.cpp.
◆ GetMotorImpulse()
|
inlinenoexcept |
Gets the current motor impulse.
Definition at line 197 of file RevoluteJoint.hpp.
◆ GetLimitState()
|
inlinenoexcept |
Gets the current limit state.
- Note
- This will be
e_inactiveLimit
unless the joint limit has been enabled.
Definition at line 192 of file RevoluteJoint.hpp.
Friends And Related Function Documentation
◆ GetJointAngle()
|
related |
Gets the current joint angle.
Definition at line 537 of file RevoluteJoint.cpp.
◆ GetAngularVelocity()
|
related |
Gets the current joint angle speed.
Definition at line 542 of file RevoluteJoint.cpp.
◆ GetMotorTorque()
|
related |
Gets the current motor torque for the given joint given the inverse time step.
Definition at line 214 of file RevoluteJoint.hpp.
◆ GetRevoluteJointConf()
|
related |
Gets the definition data for the given joint.
Definition at line 39 of file RevoluteJointConf.cpp.
The documentation for this class was generated from the following files:
- Dynamics/Joints/RevoluteJoint.hpp
- Dynamics/Joints/RevoluteJoint.cpp
- Dynamics/Joints/RevoluteJointConf.hpp