Wheel joint. More...
#include <WheelJoint.hpp>
Public Member Functions | |
WheelJoint (const WheelJointConf &def) | |
Initializing constructor. More... | |
void | Accept (JointVisitor &visitor) const override |
Accepts a visitor. More... | |
void | Accept (JointVisitor &visitor) override |
Accepts a visitor. More... | |
Length2 | GetAnchorA () const override |
Get the anchor point on body-A in world coordinates. More... | |
Length2 | GetAnchorB () const override |
Get the anchor point on body-B in world coordinates. More... | |
Momentum2 | GetLinearReaction () const override |
Get the linear reaction on body-B at the joint anchor. More... | |
AngularMomentum | GetAngularReaction () const override |
Get the angular reaction on body-B. More... | |
Length2 | GetLocalAnchorA () const |
The local anchor point relative to body A's origin. More... | |
Length2 | GetLocalAnchorB () const |
The local anchor point relative to body B's origin. More... | |
UnitVec | GetLocalAxisA () const |
The local joint axis relative to body-A. More... | |
bool | IsMotorEnabled () const noexcept |
Is the joint motor enabled? More... | |
void | EnableMotor (bool flag) |
Enable/disable the joint motor. More... | |
RotInertia | GetMotorMass () const noexcept |
Gets the computed motor mass. More... | |
void | SetMotorSpeed (AngularVelocity speed) |
Set the angular motor speed. More... | |
AngularVelocity | GetMotorSpeed () const |
Get the angular motor speed. More... | |
void | SetMaxMotorTorque (Torque torque) |
Sets the maximum motor torque. More... | |
Torque | GetMaxMotorTorque () const |
Gets the maximum motor torque. More... | |
void | SetSpringFrequency (Frequency frequency) |
Sets the spring frequency. More... | |
Frequency | GetSpringFrequency () const |
Gets the spring frequency. More... | |
void | SetSpringDampingRatio (Real ratio) |
Sets the spring damping ratio. More... | |
Real | GetSpringDampingRatio () const |
Gets the spring damping ratio. More... | |
![]() | |
virtual | ~Joint () noexcept=default |
Body * | GetBodyA () const noexcept |
Gets the first body attached to this joint. More... | |
Body * | GetBodyB () const noexcept |
Gets the second body attached to this joint. More... | |
void * | GetUserData () const noexcept |
Get the user data pointer. More... | |
void | SetUserData (void *data) noexcept |
Set the user data pointer. More... | |
bool | GetCollideConnected () const noexcept |
Gets collide connected. More... | |
virtual bool | ShiftOrigin (const Length2) |
Shifts the origin for any points stored in world coordinates. More... | |
Related Functions | |
(Note that these are not member functions.) | |
Length | GetJointTranslation (const WheelJoint &joint) noexcept |
Get the current joint translation. More... | |
AngularVelocity | GetAngularVelocity (const WheelJoint &joint) noexcept |
Get the current joint translation speed. More... | |
WheelJointConf | GetWheelJointConf (const WheelJoint &joint) noexcept |
Gets the definition data for the given joint. More... | |
![]() | |
bool | IsEnabled (const Joint &j) noexcept |
Short-cut function to determine if both bodies are enabled. More... | |
void | SetAwake (Joint &j) noexcept |
Wakes up the joined bodies. More... | |
JointCounter | GetWorldIndex (const Joint *joint) |
Gets the world index of the given joint. More... | |
JointType | GetType (const Joint &joint) noexcept |
Gets the type of the given joint. More... | |
Additional Inherited Members | |
![]() | |
enum | LimitState { e_inactiveLimit, e_atLowerLimit, e_atUpperLimit, e_equalLimits } |
Limit state. More... | |
![]() | |
static bool | IsOkay (const JointConf &def) noexcept |
Is the given definition okay. More... | |
![]() | |
Joint (const JointConf &def) | |
Initializing constructor. More... | |
Detailed Description
Wheel joint.
This joint provides two degrees of freedom: translation along an axis fixed in body A and rotation in the plane. In other words, it is a point to line constraint with a rotational motor and a linear spring/damper.
- Note
- This joint is designed for vehicle suspensions.
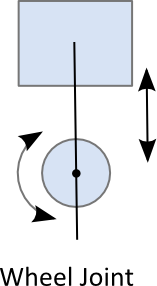
Definition at line 43 of file WheelJoint.hpp.
Constructor & Destructor Documentation
◆ WheelJoint()
playrho::d2::WheelJoint::WheelJoint | ( | const WheelJointConf & | def | ) |
Initializing constructor.
- Attention
- To create or use the joint within a world instance, call that world instance's create joint method instead of calling this constructor directly.
- See also
- World::CreateJoint
Definition at line 48 of file WheelJoint.cpp.
Member Function Documentation
◆ Accept() [1/2]
|
overridevirtual |
Accepts a visitor.
This is the Accept method definition of a "visitor design pattern" for for doing joint subclass specific types of processing for a constant joint.
Implements playrho::d2::Joint.
Definition at line 63 of file WheelJoint.cpp.
◆ Accept() [2/2]
|
overridevirtual |
Accepts a visitor.
This is the Accept method definition of a "visitor design pattern" for for doing joint subclass specific types of processing.
Implements playrho::d2::Joint.
Definition at line 68 of file WheelJoint.cpp.
◆ GetAnchorA()
|
overridevirtual |
Get the anchor point on body-A in world coordinates.
Implements playrho::d2::Joint.
Definition at line 314 of file WheelJoint.cpp.
◆ GetAnchorB()
|
overridevirtual |
Get the anchor point on body-B in world coordinates.
Implements playrho::d2::Joint.
Definition at line 319 of file WheelJoint.cpp.
◆ GetLinearReaction()
|
overridevirtual |
Get the linear reaction on body-B at the joint anchor.
Implements playrho::d2::Joint.
Definition at line 324 of file WheelJoint.cpp.
◆ GetAngularReaction()
|
inlineoverridevirtual |
Get the angular reaction on body-B.
Implements playrho::d2::Joint.
Definition at line 148 of file WheelJoint.hpp.
◆ GetLocalAnchorA()
|
inline |
The local anchor point relative to body A's origin.
Definition at line 63 of file WheelJoint.hpp.
◆ GetLocalAnchorB()
|
inline |
The local anchor point relative to body B's origin.
Definition at line 66 of file WheelJoint.hpp.
◆ GetLocalAxisA()
|
inline |
The local joint axis relative to body-A.
Definition at line 69 of file WheelJoint.hpp.
◆ IsMotorEnabled()
|
inlinenoexcept |
Is the joint motor enabled?
Definition at line 72 of file WheelJoint.hpp.
◆ EnableMotor()
void playrho::d2::WheelJoint::EnableMotor | ( | bool | flag | ) |
Enable/disable the joint motor.
Definition at line 329 of file WheelJoint.cpp.
◆ GetMotorMass()
|
inlinenoexcept |
Gets the computed motor mass.
- Note
- This is zero unless motor is enabled and either body has any rotational inertia.
Definition at line 79 of file WheelJoint.hpp.
◆ SetMotorSpeed()
void playrho::d2::WheelJoint::SetMotorSpeed | ( | AngularVelocity | speed | ) |
Set the angular motor speed.
Definition at line 341 of file WheelJoint.cpp.
◆ GetMotorSpeed()
|
inline |
Get the angular motor speed.
Definition at line 153 of file WheelJoint.hpp.
◆ SetMaxMotorTorque()
void playrho::d2::WheelJoint::SetMaxMotorTorque | ( | Torque | torque | ) |
Sets the maximum motor torque.
Definition at line 353 of file WheelJoint.cpp.
◆ GetMaxMotorTorque()
|
inline |
Gets the maximum motor torque.
Definition at line 158 of file WheelJoint.hpp.
◆ SetSpringFrequency()
|
inline |
Sets the spring frequency.
- Note
- Setting the frequency to zero disables the spring.
Definition at line 163 of file WheelJoint.hpp.
◆ GetSpringFrequency()
|
inline |
Gets the spring frequency.
Definition at line 168 of file WheelJoint.hpp.
◆ SetSpringDampingRatio()
|
inline |
Sets the spring damping ratio.
Definition at line 173 of file WheelJoint.hpp.
◆ GetSpringDampingRatio()
|
inline |
Gets the spring damping ratio.
Definition at line 178 of file WheelJoint.hpp.
Friends And Related Function Documentation
◆ GetJointTranslation()
|
related |
Get the current joint translation.
Definition at line 365 of file WheelJoint.cpp.
◆ GetAngularVelocity()
|
related |
Get the current joint translation speed.
Definition at line 374 of file WheelJoint.cpp.
◆ GetWheelJointConf()
|
related |
Gets the definition data for the given joint.
Definition at line 39 of file WheelJointConf.cpp.
The documentation for this class was generated from the following files:
- Dynamics/Joints/WheelJoint.hpp
- Dynamics/Joints/WheelJoint.cpp
- Dynamics/Joints/WheelJointConf.hpp