Collection of functions that do ray casting. More...
Functions | |
RayCastOutput | playrho::d2::RayCast (Length radius, Length2 location, const RayCastInput &input) noexcept |
Cast a ray against a circle of a given radius at the given location. More... | |
RayCastOutput | playrho::d2::RayCast (const detail::AABB< 2 > &aabb, const RayCastInput &input) noexcept |
Cast a ray against the given AABB. More... | |
RayCastOutput | playrho::d2::RayCast (const DistanceProxy &proxy, const RayCastInput &input, const Transformation &transform) noexcept |
Cast a ray against the distance proxy. More... | |
RayCastOutput | playrho::d2::RayCast (const Shape &shape, ChildCounter childIndex, const RayCastInput &input, const Transformation &transform) noexcept |
Cast a ray against the child of the given shape. More... | |
bool | playrho::d2::RayCast (const DynamicTree &tree, RayCastInput input, const DynamicTreeRayCastCB &callback) |
Cast rays against the leafs in the given tree. More... | |
bool | playrho::d2::RayCast (const DynamicTree &tree, const RayCastInput &input, FixtureRayCastCB callback) |
Ray-cast the dynamic tree for all fixtures in the path of the ray. More... | |
RayCastOutput | RayCast (const DistanceProxy &proxy, const RayCastInput &input, const Transformation &transform) noexcept |
Cast a ray against the distance proxy. More... | |
RayCastOutput | RayCast (const Shape &shape, ChildCounter childIndex, const RayCastInput &input, const Transformation &transform) noexcept |
Cast a ray against the child of the given shape. More... | |
Detailed Description
Collection of functions that do ray casting.
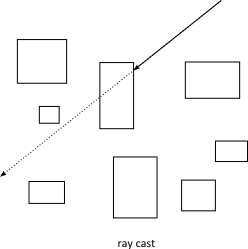
Function Documentation
◆ RayCast() [1/8]
|
noexcept |
Cast a ray against a circle of a given radius at the given location.
- Parameters
-
radius Radius of the circle. location Location in world coordinates of the circle. input Ray-cast input parameters.
- Examples
- World.cpp.
Definition at line 34 of file RayCastOutput.cpp.
◆ RayCast() [2/8]
|
noexcept |
Cast a ray against the given AABB.
- Parameters
-
aabb Axis Aligned Bounding Box. input the ray-cast input parameters.
Definition at line 70 of file RayCastOutput.cpp.
◆ RayCast() [3/8]
|
noexcept |
Cast a ray against the distance proxy.
- Parameters
-
proxy Distance-proxy object (in local coordinates). input Ray-cast input parameters. transform Transform to be applied to the distance-proxy to get world coordinates.
Definition at line 131 of file RayCastOutput.cpp.
◆ RayCast() [4/8]
|
noexcept |
Cast a ray against the child of the given shape.
- Note
- This is a convenience function for calling the ray cast against a distance-proxy.
- Parameters
-
shape Shape. childIndex Child index. input the ray-cast input parameters. transform Transform to be applied to the child of the shape.
Definition at line 228 of file RayCastOutput.cpp.
◆ RayCast() [5/8]
bool playrho::d2::RayCast | ( | const DynamicTree & | tree, |
RayCastInput | input, | ||
const DynamicTreeRayCastCB & | callback | ||
) |
Cast rays against the leafs in the given tree.
- Note
- This relies on the callback to perform an exact ray-cast in the case where the leaf node contains a shape.
- The callback also performs collision filtering.
- Performance is roughly k * log(n), where k is the number of collisions and n is the number of leaf nodes in the tree.
- Parameters
-
tree Dynamic tree to ray cast. input the ray-cast input data. The ray extends from p1
top1 + maxFraction * (p2 - p1)
.callback A callback instance function that's called for each leaf that is hit by the ray. The callback should return 0 to terminate ray casting, or greater than 0 to update the segment bounding box. Values less than zero are ignored.
- Returns
true
if terminated at the callback's request,false
otherwise.
Definition at line 234 of file RayCastOutput.cpp.
◆ RayCast() [6/8]
bool playrho::d2::RayCast | ( | const DynamicTree & | tree, |
const RayCastInput & | input, | ||
FixtureRayCastCB | callback | ||
) |
Ray-cast the dynamic tree for all fixtures in the path of the ray.
- Note
- The callback controls whether you get the closest point, any point, or n-points.
- The ray-cast ignores shapes that contain the starting point.
- Parameters
-
tree Dynamic tree to ray cast. input Ray cast input data. callback A user implemented callback function.
- Returns
true
if terminated by callback,false
otherwise.
Definition at line 293 of file RayCastOutput.cpp.
◆ RayCast() [7/8]
|
related |
Cast a ray against the distance proxy.
- Parameters
-
proxy Distance-proxy object (in local coordinates). input Ray-cast input parameters. transform Transform to be applied to the distance-proxy to get world coordinates.
- Examples
- World.cpp.
Definition at line 131 of file RayCastOutput.cpp.
◆ RayCast() [8/8]
|
related |
Cast a ray against the child of the given shape.
- Note
- This is a convenience function for calling the ray cast against a distance-proxy.
- Parameters
-
shape Shape. childIndex Child index. input the ray-cast input parameters. transform Transform to be applied to the child of the shape.
Definition at line 228 of file RayCastOutput.cpp.