#include <Shape.hpp>
Public Member Functions | |
Shape () | |
Default constructor. More... | |
template<typename T > | |
Shape (T arg) | |
Initializing constructor. More... | |
Shape (const Shape &other)=default | |
Copy constructor. More... | |
Shape (Shape &&other)=default | |
Move constructor. More... | |
Shape & | operator= (const Shape &other)=default |
Copy assignment operator. More... | |
Shape & | operator= (Shape &&other)=default |
Move assignment operator. More... | |
Friends | |
ChildCounter | GetChildCount (const Shape &shape) noexcept |
Gets the number of child primitives of the shape. More... | |
DistanceProxy | GetChild (const Shape &shape, ChildCounter index) |
Gets the "child" for the given index. More... | |
MassData | GetMassData (const Shape &shape) noexcept |
Gets the mass properties of this shape using its dimensions and density. More... | |
NonNegative< Length > | GetVertexRadius (const Shape &shape, ChildCounter idx) |
Gets the vertex radius of the indexed child of the given shape. More... | |
Real | GetFriction (const Shape &shape) noexcept |
Gets the coefficient of friction. More... | |
Real | GetRestitution (const Shape &shape) noexcept |
Gets the coefficient of restitution value of the given shape. More... | |
NonNegative< AreaDensity > | GetDensity (const Shape &shape) noexcept |
Gets the density of the given shape. More... | |
void | Transform (Shape &shape, const Mat22 &m) |
Transforms all of the given shape's vertices by the given transformation matrix. More... | |
bool | Visit (const Shape &shape, void *userData) |
Visits the given shape with the potentially non-null user data pointer. More... | |
const friend void * | GetData (const Shape &shape) noexcept |
Gets a pointer to the underlying data. More... | |
const friend std::type_info & | GetUseTypeInfo (const Shape &shape) |
Gets the type info of the use of the given shape. More... | |
void | Accept (const Shape &shape, const TypeInfoVisitor &visitor) |
Accepts a visitor. More... | |
bool | operator== (const Shape &lhs, const Shape &rhs) noexcept |
Equality operator for shape to shape comparisons. More... | |
bool | operator!= (const Shape &lhs, const Shape &rhs) noexcept |
Inequality operator for shape to shape comparisons. More... | |
Related Functions | |
(Note that these are not member functions.) | |
AABB | ComputeAABB (const Shape &shape, const Transformation &xf) noexcept |
Computes the AABB for the given shape with the given transformation. More... | |
RayCastOutput | RayCast (const Shape &shape, ChildCounter childIndex, const RayCastInput &input, const Transformation &transform) noexcept |
Cast a ray against the child of the given shape. More... | |
bool | TestPoint (const Shape &shape, Length2 point) noexcept |
Test a point for containment in the given shape. More... | |
Detailed Description
A shape is used for collision detection. You can create a shape from any supporting type. Shapes are conceptually made up of zero or more convex child shapes where each child shape is made up of zero or more vertices and an associated radius called its "vertex radius".
- Note
- This class implements polymorphism without inheritance. This is based on a technique that's described by Sean Parent in his January 2017 Norwegian Developers Conference London talk "Better Code: Runtime Polymorphism". With this implementation, different shapes types can be had by constructing instances of this class with the different types that provide the required support. Different shapes of a given type meanwhile are had by providing different values for the type.
- This data structure is 32-bytes large (on at least one 64-bit platform).
- See also
- Fixture
- https://youtu.be/QGcVXgEVMJg
- Examples
- Body.cpp, Contact.cpp, HelloWorld.cpp, and World.cpp.
Constructor & Destructor Documentation
◆ Shape() [1/4]
playrho::d2::Shape::Shape | ( | ) |
◆ Shape() [2/4]
|
inlineexplicit |
Initializing constructor.
- Parameters
-
arg Configuration value to construct a shape instance for.
- Note
- Only usable with types of values that have all of the support functions required by this class. The compiler emits errors if the given type doesn't.
- Exceptions
-
std::bad_alloc if there's a failure allocating storage.
◆ Shape() [3/4]
|
default |
Copy constructor.
◆ Shape() [4/4]
|
default |
Move constructor.
Member Function Documentation
◆ operator=() [1/2]
◆ operator=() [2/2]
Friends And Related Function Documentation
◆ GetChildCount
|
friend |
◆ GetChild
|
friend |
Gets the "child" for the given index.
- Parameters
-
shape Shape to get "child" shape of. index Index to a child element of the shape. Value must be less than the number of child primitives of the shape.
- Note
- The shape must remain in scope while the proxy is in use.
- Exceptions
-
InvalidArgument if the given index is out of range.
- See also
- GetChildCount
◆ GetMassData
◆ GetVertexRadius
|
friend |
Gets the vertex radius of the indexed child of the given shape.
This gets the radius from the vertex that the shape's "skin" should extend outward by. While any edges - line segments between multiple vertices - are straight, corners between them (the vertices) are rounded and treated as rounded. Shapes with larger vertex radiuses compared to edge lengths therefore will be more prone to rolling or having other shapes more prone to roll off of them. Here's an image of a shape configured via a PolygonShapeConf
with it's skin drawn:
- Parameters
-
shape Shape to get child's vertex radius for. idx Child index to get vertex radius for.
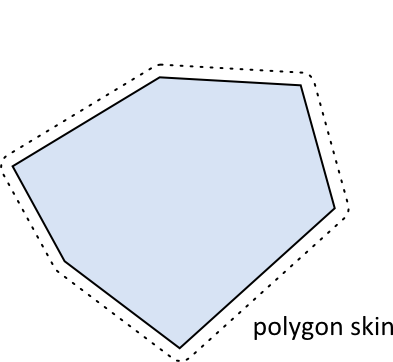
- Note
- This must be a non-negative value.
- See also
- UseVertexRadius
- Exceptions
-
InvalidArgument if the child index is not less than the child count.
◆ GetFriction
◆ GetRestitution
◆ GetDensity
|
friend |
◆ Transform
Transforms all of the given shape's vertices by the given transformation matrix.
- Note
- This may throw
std::bad_alloc
or any exception that's thrown by the constructor for the model's underlying data type.
- Exceptions
-
std::bad_alloc if there's a failure allocating storage.
◆ Visit
|
friend |
Visits the given shape with the potentially non-null user data pointer.
◆ GetData
|
friend |
◆ GetUseTypeInfo
|
friend |
◆ Accept
|
friend |
Accepts a visitor.
This is the "accept" method definition of a "visitor design pattern" for doing shape configuration specific types of processing for a constant shape.
◆ operator==
◆ operator!=
◆ ComputeAABB()
|
related |
The documentation for this class was generated from the following files:
- Collision/Shapes/Shape.hpp
- Collision/AABB.hpp
- Collision/RayCastOutput.hpp
- Collision/Shapes/Shape.cpp