Pulley joint. More...
#include <PulleyJoint.hpp>
Public Member Functions | |
PulleyJoint (const PulleyJointConf &data) | |
Initializing constructor. More... | |
void | Accept (JointVisitor &visitor) const override |
Accepts a visitor. More... | |
void | Accept (JointVisitor &visitor) override |
Accepts a visitor. More... | |
Length2 | GetAnchorA () const override |
Get the anchor point on body-A in world coordinates. More... | |
Length2 | GetAnchorB () const override |
Get the anchor point on body-B in world coordinates. More... | |
Momentum2 | GetLinearReaction () const override |
Get the linear reaction on body-B at the joint anchor. More... | |
AngularMomentum | GetAngularReaction () const override |
Get the angular reaction on body-B. More... | |
bool | ShiftOrigin (const Length2 newOrigin) override |
Shifts the origin for any points stored in world coordinates. More... | |
Length2 | GetLocalAnchorA () const noexcept |
Gets the local anchor A. More... | |
Length2 | GetLocalAnchorB () const noexcept |
Gets the local anchor B. More... | |
Length2 | GetGroundAnchorA () const noexcept |
Get the first ground anchor. More... | |
Length2 | GetGroundAnchorB () const noexcept |
Get the second ground anchor. More... | |
Length | GetLengthA () const noexcept |
Get the current length of the segment attached to body-A. More... | |
Length | GetLengthB () const noexcept |
Get the current length of the segment attached to body-B. More... | |
Real | GetRatio () const noexcept |
Get the pulley ratio. More... | |
![]() | |
virtual | ~Joint () noexcept=default |
Body * | GetBodyA () const noexcept |
Gets the first body attached to this joint. More... | |
Body * | GetBodyB () const noexcept |
Gets the second body attached to this joint. More... | |
void * | GetUserData () const noexcept |
Get the user data pointer. More... | |
void | SetUserData (void *data) noexcept |
Set the user data pointer. More... | |
bool | GetCollideConnected () const noexcept |
Gets collide connected. More... | |
Related Functions | |
(Note that these are not member functions.) | |
Length | GetCurrentLengthA (const PulleyJoint &joint) |
Get the current length of the segment attached to body-A. More... | |
Length | GetCurrentLengthB (const PulleyJoint &joint) |
Get the current length of the segment attached to body-B. More... | |
PulleyJointConf | GetPulleyJointConf (const PulleyJoint &joint) noexcept |
Gets the definition data for the given joint. More... | |
![]() | |
bool | IsEnabled (const Joint &j) noexcept |
Short-cut function to determine if both bodies are enabled. More... | |
void | SetAwake (Joint &j) noexcept |
Wakes up the joined bodies. More... | |
JointCounter | GetWorldIndex (const Joint *joint) |
Gets the world index of the given joint. More... | |
JointType | GetType (const Joint &joint) noexcept |
Gets the type of the given joint. More... | |
Additional Inherited Members | |
![]() | |
enum | LimitState { e_inactiveLimit, e_atLowerLimit, e_atUpperLimit, e_equalLimits } |
Limit state. More... | |
![]() | |
static bool | IsOkay (const JointConf &def) noexcept |
Is the given definition okay. More... | |
![]() | |
Joint (const JointConf &def) | |
Initializing constructor. More... | |
Detailed Description
Pulley joint.
The pulley joint is connected to two bodies and two fixed ground points. The pulley supports a ratio such that: length1 + ratio * length2 <= constant
.
- Note
- The force transmitted is scaled by the ratio.
- Warning
- the pulley joint can get a bit squirrelly by itself. They often work better when combined with prismatic joints. You should also cover the the anchor points with static shapes to prevent one side from going to zero length.
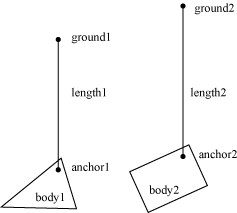
Definition at line 47 of file PulleyJoint.hpp.
Constructor & Destructor Documentation
◆ PulleyJoint()
playrho::d2::PulleyJoint::PulleyJoint | ( | const PulleyJointConf & | data | ) |
Initializing constructor.
- Attention
- To create or use the joint within a world instance, call that world instance's create joint method instead of calling this constructor directly.
- See also
- World::CreateJoint
Definition at line 44 of file PulleyJoint.cpp.
Member Function Documentation
◆ Accept() [1/2]
|
overridevirtual |
Accepts a visitor.
This is the Accept method definition of a "visitor design pattern" for for doing joint subclass specific types of processing for a constant joint.
Implements playrho::d2::Joint.
Definition at line 58 of file PulleyJoint.cpp.
◆ Accept() [2/2]
|
overridevirtual |
Accepts a visitor.
This is the Accept method definition of a "visitor design pattern" for for doing joint subclass specific types of processing.
Implements playrho::d2::Joint.
Definition at line 63 of file PulleyJoint.cpp.
◆ GetAnchorA()
|
overridevirtual |
Get the anchor point on body-A in world coordinates.
Implements playrho::d2::Joint.
Definition at line 218 of file PulleyJoint.cpp.
◆ GetAnchorB()
|
overridevirtual |
Get the anchor point on body-B in world coordinates.
Implements playrho::d2::Joint.
Definition at line 223 of file PulleyJoint.cpp.
◆ GetLinearReaction()
|
overridevirtual |
Get the linear reaction on body-B at the joint anchor.
Implements playrho::d2::Joint.
Definition at line 228 of file PulleyJoint.cpp.
◆ GetAngularReaction()
|
overridevirtual |
Get the angular reaction on body-B.
Implements playrho::d2::Joint.
Definition at line 233 of file PulleyJoint.cpp.
◆ ShiftOrigin()
|
overridevirtual |
Shifts the origin for any points stored in world coordinates.
- Returns
true
if shift done,false
otherwise.
Reimplemented from playrho::d2::Joint.
Definition at line 238 of file PulleyJoint.cpp.
◆ GetLocalAnchorA()
|
inlinenoexcept |
Gets the local anchor A.
Definition at line 115 of file PulleyJoint.hpp.
◆ GetLocalAnchorB()
|
inlinenoexcept |
Gets the local anchor B.
Definition at line 120 of file PulleyJoint.hpp.
◆ GetGroundAnchorA()
|
inlinenoexcept |
Get the first ground anchor.
Definition at line 125 of file PulleyJoint.hpp.
◆ GetGroundAnchorB()
|
inlinenoexcept |
Get the second ground anchor.
Definition at line 130 of file PulleyJoint.hpp.
◆ GetLengthA()
|
inlinenoexcept |
Get the current length of the segment attached to body-A.
Definition at line 135 of file PulleyJoint.hpp.
◆ GetLengthB()
|
inlinenoexcept |
Get the current length of the segment attached to body-B.
Definition at line 140 of file PulleyJoint.hpp.
◆ GetRatio()
|
inlinenoexcept |
Get the pulley ratio.
Definition at line 145 of file PulleyJoint.hpp.
Friends And Related Function Documentation
◆ GetCurrentLengthA()
|
related |
Get the current length of the segment attached to body-A.
Definition at line 245 of file PulleyJoint.cpp.
◆ GetCurrentLengthB()
|
related |
Get the current length of the segment attached to body-B.
Definition at line 251 of file PulleyJoint.cpp.
◆ GetPulleyJointConf()
|
related |
Gets the definition data for the given joint.
Definition at line 43 of file PulleyJointConf.cpp.
The documentation for this class was generated from the following files:
- Dynamics/Joints/PulleyJoint.hpp
- Dynamics/Joints/PulleyJoint.cpp
- Dynamics/Joints/PulleyJointConf.hpp